I like that in powershell I can use Add-Type to mix in c# code but it ends up being a bit awkward to use c# on the fly – I have to write a big string containing the c# code I want to run, and there’s no autocompletion support for that. what i’d really like is a repl.
these two projects take two very different approaches to a c# repl:
dotnet-repl is built on top of dotnet interactive, the same technology which powers polyglot notebooks in vscode. i like the idea of being able to shift between multiple languages depending on what I’m trying to do, but i also cannot stand the keybindings that dotnet-repl uses ๐ they are too different from normal shell keybindings.
i find CSharpRepl to be a lot nicer to interact with but it is c#-only and not embeddable inside of other applications.
however!! the author of CSharpRepl has published the fancy readline implementation that CSharpRepl uses, as a separate package: PrettyPrompt.
so i thought: what if i glued these together? this is very much a work in progress but i think it’s pretty neat so far!
https://github.com/vivlim/dotnet-repl
I think having easy access to dotnet interactive opens up a ton of possibilities. you can do all of this in polyglot notebooks but there’s a little more setup involved there. it’s a bit less convenient than a repl you can quickly launch in a working directory.
for an example, I’ll retrieve the contents of my clipboard in powershell, move into c#, make each word uppercase using linq, and take the resulting string back into powershell. here’s the powershell kernel, running Get-Clipboard (i copied another paragraph from this post)

i can access that from C# using the variable sharing magic command:
pwsh> $clip = Get-Clipboard
pwsh> #!csharp
csharp> #!share --from pwsh clip --as clip
Code language: PHP (php)
and manipulate that from c#, with completions. i usually find linq delegates more ergonomic to write than the powershell equivalent with select-object:
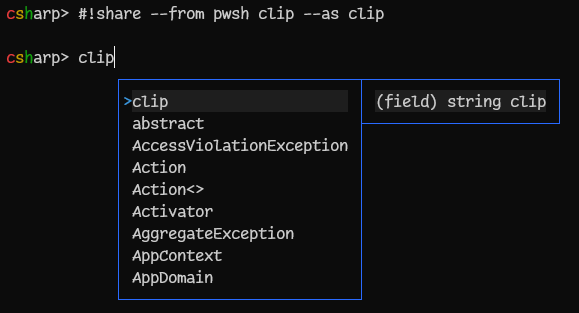
The c# kernel provides descriptions for the completion items, which are shown during selection:
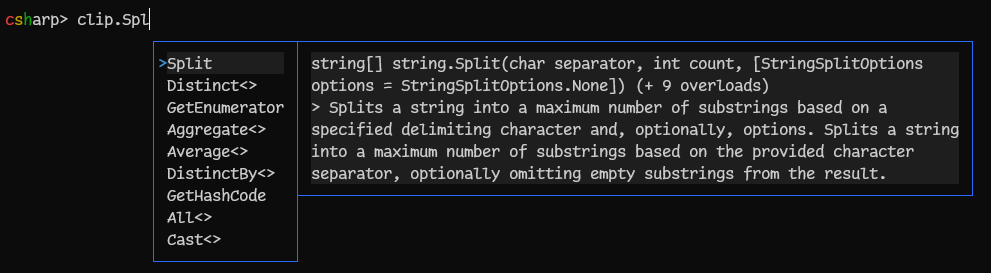

if i assign that to a variable I can get it from powershell.
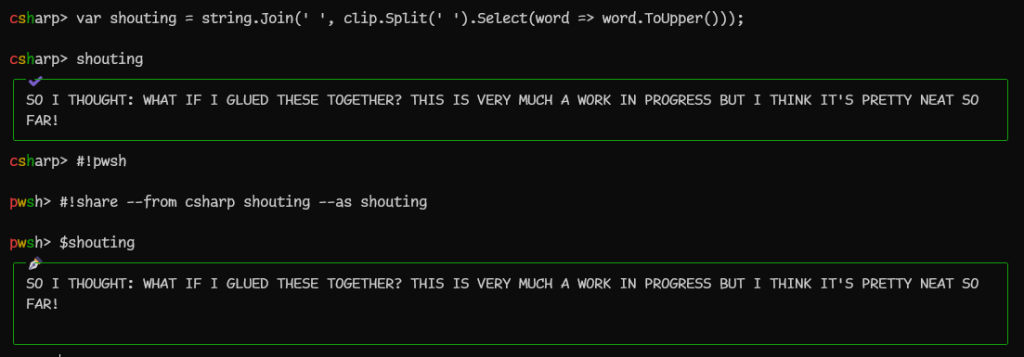
another feature of PrettyPrompt is that I can implement custom descriptions, so if I’m in the pwsh kernel and the completion item is a file path I can do something like, list the directory contents in the tooltip. this seems like it’ll make using the pwsh kernel pretty convenient
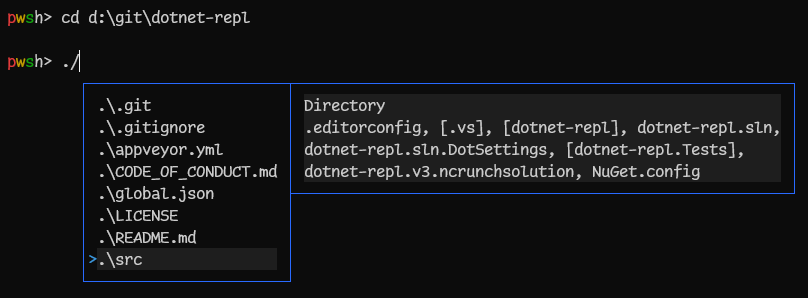
i can also (attempt to) pass objects around by reference, and manipulate them directly. for example if i use Get-ChildItem (ls) in powershell, the resulting items contain many properties and methods related to the file. I can then access that directly in c# without any serialization or deserialization taking place, and read properties / call methods on it!
pwsh> $readme = get-childitem ./README.md
pwsh> #!csharp
csharp> #!set --value @pwsh:readme --byref --name readme
csharp> readme.LastAccessTimeUtc // returns 2024-09-08 03:41:55Z
csharp> var f = readme.OpenRead();
csharp> var sr = new System.IO.StreamReader(f);
csharp> var line = await sr.ReadLineAsync(); // note i'm able to await an async method here!
csharp> line
โญโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโฎ
โ # dotnet-repl โ
โฐโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโฏ
csharp>
Code language: PHP (php)
I want to add some aliases (slash commands?) for common tasks that it’d be nice to be able to do easily, like:
- read and write clipboard
- split a string into an array of lines
- join an array of lines
- (de)serialize json, yaml, xml, csv
- filter lines by regex and extract groups
those are all things that can be done with various cli tools but it’d be nice to be able to do them in one place with a consistent interface and without needing to lossily transform data to and from text. the idea of adding more kernels to make it easy to work on other domains with rich language support also seems interesting. (maybe a bash kernel that exposes bash_completions? a regex kernel? remote systemd unit management kernel?)
Leave a Reply